1. Install Django
- Download the official release. (pip install is a good choice)
- Once all the files finish downloading, we need to make sure our local Python installation is aware that Django exists on our machine. There are a couple ways to go about that, but a symbolic link to your Python site packages directory is probably the easiest.
- Assuming you’re on a *nix system, this line will do the trick:
ln -s /home/username/.local/lib/python2.7/site-packages/django /usr/lib/python2.7/dist-packages/django
- If you don’t know where your Python site directory is, here’s a handy bit of Python that will tell you:
python -c "from distutils.sysconfig import get_python_lib; print get_python_lib()"
- If you’re on Windows, the easiest thing to do is add Django to your PythonPath environment variable. On Windows, you can define environment variables in the Control Panel.
Django installation docs suggest creating a symbolic link to the file /home/username/.local/lib/python2.7/site-packages/django/bin/django-admin.py in a directory on your system path, such as /usr/local/bin. Just paste this code in your shell.
ln -s /home/username/.local/lib/python2.7/site-packages/django/bin/django-admin.py /usr/local/bin
- Now that Django is installed and Python knows where it lives, we’re ready to get started.
2. Set up your first project
OK, let’s get started. From the command line, switch to your web development directory. Something like this:
cd ~/sites/dev
Now we’re going to run the django-admin tool we mentioned earlier. If you created the symlink, you don’t need the full path, but if you didn’t or if it doesn't work, here’s the code:
python /home/username/.local/lib/python2.7/site-packages/django/bin/django-admin.py startproject ProjectName
Now cd over to the new folder:
cd ~/sites/dev/ProjectName
This is going to be our project folder into which we will add various apps.
- django-admin.py is a script that will create the directories and files for you. You should now have a directory structure which looks like this:
ProjectName
├───manage.py
└───ProjectName
|__ settings.py
|__ urls.py
|__ wsgi.py
|__ __init__.py
3. Changing settings
The next step is to fill out our project settings file. Fire up your favorite text editor and open up the settings.py file inside the “
ProjectName/ProjectName” directory.
There's a lot of different database software that can store data for your site. We'll use the default one, sqlite3.
This is already set up in this part of your
ProjectName/settings.py file:
DATABASES = {
'default': {
'ENGINE': 'django.db.backends.sqlite3',
'NAME': os.path.join(BASE_DIR, 'db.sqlite3'),
}
}
To create a database for our site / app, let's run the following in the console:
python manage.py migrate
(we need to be in the
ProjectName directory that contains the
manage.py file). If that goes well, you should see something like this:
(myvenv) ~/ProjectName$ python manage.py migrate
Operations to perform:
Apply all migrations: auth, admin, contenttypes, sessions
Running migrations:
Rendering model states... DONE
Applying contenttypes.0001_initial... OK
Applying auth.0001_initial... OK
Applying admin.0001_initial... OK
Applying admin.0002_logentry_remove_auto_add... OK
Applying contenttypes.0002_remove_content_type_name... OK
Applying auth.0002_alter_permission_name_max_length... OK
Applying auth.0003_alter_user_email_max_length... OK
Applying auth.0004_alter_user_username_opts... OK
Applying auth.0005_alter_user_last_login_null... OK
Applying auth.0006_require_contenttypes_0002... OK
Applying auth.0007_alter_validators_add_error_messages... OK
Applying sessions.0001_initial... OK
The other settings are well documented in the settings.py file and we can skip over most of them for now.
And we're done! Time to start the web server and see if our website is working!
You need to be in the directory that contains the manage.py file (the ProjectName directory). In the console, we can start the web server by running
python manage.py runserver:
If you are on Windows and this fails with UnicodeDecodeError, use this command instead:
(myvenv) ~/ProjectName$ python manage.py runserver 0:8000
Now all you need to do is check that your website is running. Open your browser (Firefox, Chrome, Safari, Internet Explorer or whatever you use) and enter the address:
http://127.0.0.1:8000/
The web server will take over your command prompt until you stop it. To stop the web server, switch back to the window in which it's running and pressing CTRL+C - Control and C buttons together (on Windows, you might have to press Ctrl+Break).
Congratulations! You've just created your first website and run it using a web server!
One more thing before we finish with settings.py, here’s a handy trick for the template directories. I generally keep all my templates in a folder named “templates” within my project folder (in this case, “ProjectName”). But I generally move between development and live servers quite a bit and I hate having to change the path to the templates folder. This trick takes care of that:
import os.path
TEMPLATE_DIRS = (
os.path.join(os.path.dirname(__file__), 'templates'),
)
Instead of hard coding the path to our templates folder this is dynamic — and it showcases how easy it is to tweak Django using Python. We just import the os.path Python module and then find the path to the directory where settings.py is and then appends ‘templates’ to that path.
Now when we push the site live, there’s no need to change the settings.py file.
4. Creating an application
$ python manage.py startapp AppName
You will notice that a new blog directory is created and it contains a number of files now. Our directories and files in our project should look like this:
djangogirls
├── blog
│ ├── __init__.py
│ ├── admin.py
│ ├── apps.py
│ ├── migrations
│ │ └── __init__.py
│ ├── models.py
│ ├── tests.py
│ └── views.py
├── db.sqlite3
├── manage.py
└── mysite
├── __init__.py
├── settings.py
├── urls.py
└── wsgi.py
After creating an application we also need to tell Django that it should use it. We do that in the file
ProjectName/settings.py. We need to find
INSTALLED_APPS and add a line containing '
AppName'. So the final product should look like this:
INSTALLED_APPS = (
'django.contrib.admin',
'django.contrib.auth',
'django.contrib.contenttypes',
'django.contrib.sessions',
'django.contrib.messages',
'django.contrib.staticfiles',
'AppName',
)
In the AppName/models.py file we define all objects called Models - this is a place in which we will define our contents.
For Example, if we want to create blog posts, let's open AppName/models.py, remove everything from it and write code like this.
from django.db import models
from django.utils import timezone
class ObjectName(models.Model):
author = models.ForeignKey('auth.User')
title = models.CharField(max_length=200)
text = models.TextField()
created_date = models.DateTimeField(
default=timezone.now)
published_date = models.DateTimeField(
blank=True, null=True)
def publish(self):
self.published_date = timezone.now()
self.save()
def __str__(self):
return self.title
Double-check that you use two underscore characters (_) on each side of str. This convention is used frequently in Python and sometimes we also call them "dunder" (short for "double-underscore").
Let’s step through the code line by line and we’ll talk about what’s going on.
class ObjectName(models.Model): - this line defines our model (it is an object).
class is a special keyword that indicates that we are defining an object.
ObjectName is the name of our model. We can give it a different name (but we must avoid special characters and whitespaces). Always start a class name with an uppercase letter.
models.Model means that the ObjectName is a Django Model, so Django knows that it should be saved in the database.
5. Create tables for models in your database
The last step here is to add our new model to our database. First we have to make Django know that we have some changes in our model (we have just created it!). Go to your console window and type
python manage.py makemigrations AppName
It will look like this:
(myvenv) ~/ProjectName$ python manage.py makemigrations AppName
Migrations for 'AppName':
0001_initial.py:
- Create model Post
Django prepared for us a migration file that we have to apply now to our database.
Type python manage.py migrate AppName and the output should be:
(myvenv) ~/ProjectName$ python manage.py migrate AppName
Operations to perform:
Apply all migrations: blog
Running migrations:
Rendering model states... DONE
Applying blog.0001_initial... OK
Hurray! Our model is now in our database!
6. Django admin
To add, edit and delete posts we've just modeled, we will use Django admin.
Let's open the AppName/admin.py file and replace its content with this:
from django.contrib import admin
from .models import ObjectName
admin.site.register(ObjectName)
As you can see, we import (include) the Object model defined in the previous chapter. To make our model visible on the admin page, we need to register the model with admin.site.register(ObjectName).
OK, time to look at our Object model. Remember to run python manage.py runserver in the console to run the web server. Go to the browser and type the address http://127.0.0.1:8000/admin/ You will see a login page like this:
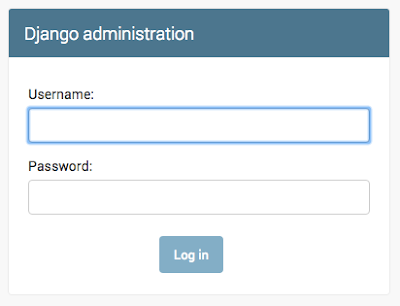
To log in, you need to create a superuser - a user which has control over everything on the site. Go back to the command-line and type python manage.py createsuperuser, and press enter. When prompted, type your username (lowercase, no spaces), email address, and password.
(myvenv) ~/ProjectName$ python manage.py createsuperuser
Username: admin
Email address: admin@admin.com
Password:
Password (again):
Superuser created successfully.
Return to your browser. Log in with the superuser's credentials you chose; you should see the Django admin dashboard.